How To Loop Through A Set In Python
Watch Now This tutorial has a related video form created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: For Loops in Python (Definite Iteration)
This tutorial will show you how to perform definite iteration with a Python for
loop.
In the previous tutorial in this introductory series, you learned the following:
- Repetitive execution of the same block of lawmaking over and over is referred to as iteration.
- There are 2 types of iteration:
- Definite iteration, in which the number of repetitions is specified explicitly in accelerate
- Indefinite iteration, in which the code block executes until some condition is met
- In Python, indefinite iteration is performed with a
while
loop.
Here's what you'll cover in this tutorial:
-
You'll start with a comparison of some different paradigms used by programming languages to implement definite iteration.
-
So you lot will learn about iterables and iterators, two concepts that form the footing of definite iteration in Python.
-
Finally, you'll tie it all together and learn nigh Python's
for
loops.
A Survey of Definite Iteration in Programming
Definite iteration loops are ofttimes referred to as for
loops because for
is the keyword that is used to innovate them in almost all programming languages, including Python.
Historically, programming languages have offered a few contrasted flavors of for
loop. These are briefly described in the following sections.
Numeric Range Loop
The about basic for
loop is a simple numeric range statement with start and end values. The verbal format varies depending on the language but typically looks something like this:
for i = ane to ten < loop body >
Here, the body of the loop is executed x times. The variable i
assumes the value 1
on the get-go iteration, 2
on the second, so on. This sort of for
loop is used in the languages Bones, Algol, and Pascal.
Three-Expression Loop
Some other grade of for
loop popularized by the C programming linguistic communication contains three parts:
- An initialization
- An expression specifying an catastrophe condition
- An action to be performed at the end of each iteration.
This type of loop has the following form:
for ( i = one ; i <= 10 ; i ++ ) < loop body >
This loop is interpreted as follows:
- Initialize
i
to1
. - Continue looping as long every bit
i <= x
. - Increase
i
by1
afterward each loop iteration.
Three-expression for
loops are popular considering the expressions specified for the three parts can be nearly anything, so this has quite a scrap more than flexibility than the simpler numeric range course shown in a higher place. These for
loops are also featured in the C++, Java, PHP, and Perl languages.
Collection-Based or Iterator-Based Loop
This type of loop iterates over a collection of objects, rather than specifying numeric values or conditions:
for i in < collection > < loop torso >
Each fourth dimension through the loop, the variable i
takes on the value of the next object in <collection>
. This type of for
loop is arguably the about generalized and abstract. Perl and PHP also support this blazon of loop, but it is introduced by the keyword foreach
instead of for
.
The Python for
Loop
Of the loop types listed above, Python only implements the concluding: drove-based iteration. At offset blush, that may seem similar a raw deal, simply rest assured that Python'southward implementation of definite iteration is and then versatile that you won't cease up feeling cheated!
Shortly, you lot'll dig into the guts of Python's for
loop in detail. Just for at present, let'south start with a quick prototype and example, just to become acquainted.
Python's for
loop looks like this:
for < var > in < iterable > : < statement ( s ) >
<iterable>
is a collection of objects—for instance, a list or tuple. The <statement(s)>
in the loop body are denoted by indentation, as with all Python command structures, and are executed in one case for each particular in <iterable>
. The loop variable <var>
takes on the value of the next element in <iterable>
each time through the loop.
Here is a representative example:
>>>
>>> a = [ 'foo' , 'bar' , 'baz' ] >>> for i in a : ... print ( i ) ... foo bar baz
In this example, <iterable>
is the list a
, and <var>
is the variable i
. Each time through the loop, i
takes on a successive item in a
, then impress()
displays the values 'foo'
, 'bar'
, and 'baz'
, respectively. A for
loop like this is the Pythonic way to procedure the items in an iterable.
Merely what exactly is an iterable? Before examining for
loops farther, it volition be beneficial to delve more deeply into what iterables are in Python.
Iterables
In Python, iterable ways an object can be used in iteration. The term is used every bit:
- An adjective: An object may be described every bit iterable.
- A noun: An object may be characterized equally an iterable.
If an object is iterable, it can be passed to the built-in Python part iter()
, which returns something chosen an iterator. Yes, the terminology gets a flake repetitive. Hang in there. It all works out in the end.
Each of the objects in the following case is an iterable and returns some blazon of iterator when passed to iter()
:
>>>
>>> iter ( 'foobar' ) # String <str_iterator object at 0x036E2750> >>> iter ([ 'foo' , 'bar' , 'baz' ]) # List <list_iterator object at 0x036E27D0> >>> iter (( 'foo' , 'bar' , 'baz' )) # Tuple <tuple_iterator object at 0x036E27F0> >>> iter ({ 'foo' , 'bar' , 'baz' }) # Set <set_iterator object at 0x036DEA08> >>> iter ({ 'foo' : 1 , 'bar' : 2 , 'baz' : three }) # Dict <dict_keyiterator object at 0x036DD990>
These object types, on the other hand, aren't iterable:
>>>
>>> iter ( 42 ) # Integer Traceback (most recent call last): File "<pyshell#26>", line 1, in <module> iter ( 42 ) TypeError: 'int' object is non iterable >>> iter ( 3.i ) # Bladder Traceback (most recent telephone call last): File "<pyshell#27>", line ane, in <module> iter ( 3.i ) TypeError: 'bladder' object is not iterable >>> iter ( len ) # Built-in function Traceback (virtually contempo call last): File "<pyshell#28>", line ane, in <module> iter ( len ) TypeError: 'builtin_function_or_method' object is not iterable
All the data types you have encountered so far that are collection or container types are iterable. These include the string, list, tuple, dict, ready, and frozenset types.
But these are by no ways the only types that you tin iterate over. Many objects that are built into Python or defined in modules are designed to exist iterable. For instance, open files in Python are iterable. As you will meet before long in the tutorial on file I/O, iterating over an open file object reads data from the file.
In fact, almost any object in Python can be fabricated iterable. Even user-defined objects tin be designed in such a manner that they tin can be iterated over. (Yous will notice out how that is done in the upcoming article on object-oriented programming.)
Iterators
Okay, at present you know what it means for an object to be iterable, and you know how to use iter()
to obtain an iterator from it. Once you lot've got an iterator, what can yous do with it?
An iterator is substantially a value producer that yields successive values from its associated iterable object. The built-in office adjacent()
is used to obtain the next value from in iterator.
Here is an example using the aforementioned list as above:
>>>
>>> a = [ 'foo' , 'bar' , 'baz' ] >>> itr = iter ( a ) >>> itr <list_iterator object at 0x031EFD10> >>> next ( itr ) 'foo' >>> next ( itr ) 'bar' >>> next ( itr ) 'baz'
In this example, a
is an iterable list and itr
is the associated iterator, obtained with iter()
. Each next(itr)
telephone call obtains the next value from itr
.
Notice how an iterator retains its country internally. It knows which values have been obtained already, so when you lot call next()
, it knows what value to return next.
What happens when the iterator runs out of values? Permit's make i more next()
call on the iterator higher up:
>>>
>>> next ( itr ) Traceback (most contempo call concluding): File "<pyshell#10>", line 1, in <module> side by side ( itr ) StopIteration
If all the values from an iterator have been returned already, a subsequent adjacent()
call raises a StopIteration
exception. Any farther attempts to obtain values from the iterator will fail.
You can but obtain values from an iterator in i management. You tin't go backward. There is no prev()
function. But you lot can define 2 contained iterators on the same iterable object:
>>>
>>> a ['foo', 'bar', 'baz'] >>> itr1 = iter ( a ) >>> itr2 = iter ( a ) >>> side by side ( itr1 ) 'foo' >>> side by side ( itr1 ) 'bar' >>> adjacent ( itr1 ) 'baz' >>> next ( itr2 ) 'foo'
Even when iterator itr1
is already at the end of the list, itr2
is yet at the beginning. Each iterator maintains its ain internal land, contained of the other.
If you want to grab all the values from an iterator at in one case, you can employ the built-in listing()
function. Amongst other possible uses, list()
takes an iterator as its argument, and returns a list consisting of all the values that the iterator yielded:
>>>
>>> a = [ 'foo' , 'bar' , 'baz' ] >>> itr = iter ( a ) >>> list ( itr ) ['foo', 'bar', 'baz']
Similarly, the built-in tuple()
and ready()
functions return a tuple and a set, respectively, from all the values an iterator yields:
>>>
>>> a = [ 'foo' , 'bar' , 'baz' ] >>> itr = iter ( a ) >>> tuple ( itr ) ('foo', 'bar', 'baz') >>> itr = iter ( a ) >>> ready ( itr ) {'baz', 'foo', 'bar'}
It isn't necessarily advised to make a addiction of this. Part of the elegance of iterators is that they are "lazy." That means that when y'all create an iterator, it doesn't generate all the items it can yield just then. Information technology waits until you ask for them with next()
. Items are not created until they are requested.
When you use listing()
, tuple()
, or the like, y'all are forcing the iterator to generate all its values at once, so they tin can all be returned. If the total number of objects the iterator returns is very large, that may take a long fourth dimension.
In fact, it is possible to create an iterator in Python that returns an endless serial of objects using generator functions and itertools
. If you endeavor to grab all the values at once from an endless iterator, the plan will hang.
The Guts of the Python for
Loop
You now have been introduced to all the concepts you lot need to fully understand how Python's for
loop works. Before proceeding, let's review the relevant terms:
Term | Meaning |
---|---|
Iteration | The procedure of looping through the objects or items in a collection |
Iterable | An object (or the describing word used to describe an object) that tin can exist iterated over |
Iterator | The object that produces successive items or values from its associated iterable |
iter() | The built-in function used to obtain an iterator from an iterable |
At present, consider over again the simple for
loop presented at the start of this tutorial:
>>>
>>> a = [ 'foo' , 'bar' , 'baz' ] >>> for i in a : ... print ( i ) ... foo bar baz
This loop can be described entirely in terms of the concepts you have merely learned about. To carry out the iteration this for
loop describes, Python does the following:
- Calls
iter()
to obtain an iterator fora
- Calls
adjacent()
repeatedly to obtain each item from the iterator in turn - Terminates the loop when
next()
raises theStopIteration
exception
The loop trunk is executed once for each item next()
returns, with loop variable i
set up to the given item for each iteration.
This sequence of events is summarized in the following diagram:
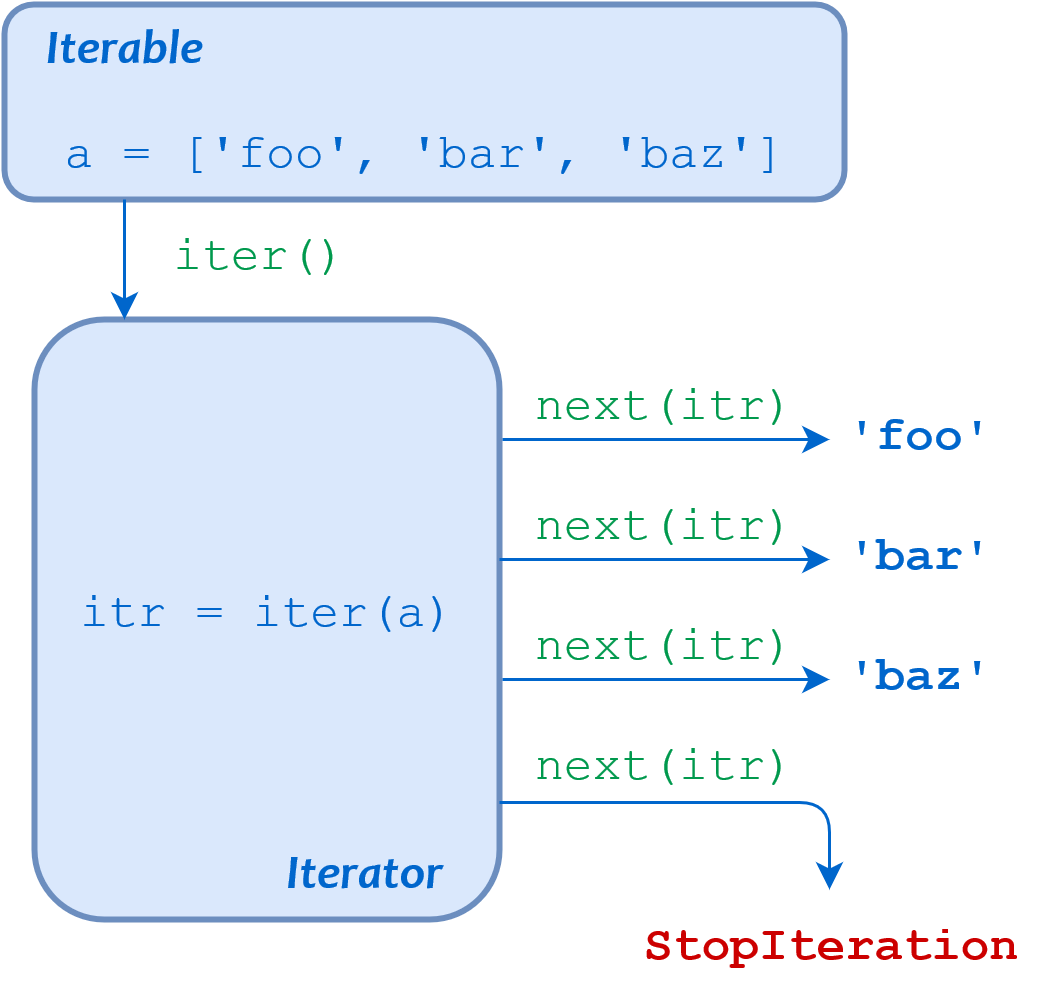
Perhaps this seems like a lot of unnecessary monkey concern, merely the benefit is substantial. Python treats looping over all iterables in exactly this way, and in Python, iterables and iterators abound:
-
Many built-in and library objects are iterable.
-
There is a Standard Library module called
itertools
containing many functions that return iterables. -
User-defined objects created with Python's object-oriented capability can be made to exist iterable.
-
Python features a construct chosen a generator that allows you to create your own iterator in a simple, straightforward way.
Y'all will discover more near all the above throughout this serial. They can all be the target of a for
loop, and the syntax is the same across the board. It's elegant in its simplicity and eminently versatile.
Iterating Through a Lexicon
You saw earlier that an iterator can be obtained from a dictionary with iter()
, so you know dictionaries must be iterable. What happens when yous loop through a lexicon? Allow'due south see:
>>>
>>> d = { 'foo' : 1 , 'bar' : 2 , 'baz' : 3 } >>> for thousand in d : ... print ( one thousand ) ... foo bar baz
Every bit you can see, when a for
loop iterates through a dictionary, the loop variable is assigned to the lexicon's keys.
To access the dictionary values within the loop, yous tin can make a lexicon reference using the key as usual:
>>>
>>> for yard in d : ... impress ( d [ k ]) ... 1 2 3
You can also iterate through a lexicon's values directly by using .values()
:
>>>
>>> for v in d . values (): ... print ( v ) ... 1 2 3
In fact, you lot tin iterate through both the keys and values of a dictionary simultaneously. That is because the loop variable of a for
loop isn't limited to only a single variable. It can also exist a tuple, in which case the assignments are made from the items in the iterable using packing and unpacking, just as with an assignment statement:
>>>
>>> i , j = ( 1 , 2 ) >>> impress ( i , j ) 1 2 >>> for i , j in [( i , two ), ( 3 , four ), ( five , 6 )]: ... print ( i , j ) ... 1 ii 3 4 5 6
As noted in the tutorial on Python dictionaries, the lexicon method .items()
effectively returns a list of key/value pairs as tuples:
>>>
>>> d = { 'foo' : 1 , 'bar' : ii , 'baz' : 3 } >>> d . items () dict_items([('foo', one), ('bar', 2), ('baz', iii)])
Thus, the Pythonic style to iterate through a dictionary accessing both the keys and values looks like this:
>>>
>>> d = { 'foo' : one , 'bar' : 2 , 'baz' : 3 } >>> for yard , v in d . items (): ... print ( 'chiliad =' , thousand , ', v =' , five ) ... grand = foo , v = i k = bar , v = 2 1000 = baz , v = three
The range()
Function
In the first section of this tutorial, yous saw a blazon of for
loop chosen a numeric range loop, in which starting and ending numeric values are specified. Although this grade of for
loop isn't directly congenital into Python, it is hands arrived at.
For case, if you wanted to iterate through the values from 0
to 4
, you could just exercise this:
>>>
>>> for northward in ( 0 , 1 , 2 , 3 , 4 ): ... print ( n ) ... 0 1 2 3 4
This solution isn't too bad when at that place are only a few numbers. But if the number range were much larger, it would go tedious pretty quickly.
Happily, Python provides a better option—the built-in range()
function, which returns an iterable that yields a sequence of integers.
range(<end>)
returns an iterable that yields integers starting with 0
, up to simply not including <terminate>
:
>>>
>>> 10 = range ( 5 ) >>> x range(0, 5) >>> blazon ( x ) <course 'range'>
Note that range()
returns an object of form range
, not a list or tuple of the values. Because a range
object is an iterable, you can obtain the values by iterating over them with a for
loop:
>>>
>>> for due north in x : ... print ( n ) ... 0 one ii 3 4
You lot could also snag all the values at one time with list()
or tuple()
. In a REPL session, that can be a user-friendly way to quickly display what the values are:
>>>
>>> listing ( x ) [0, 1, two, 3, 4] >>> tuple ( x ) (0, 1, 2, iii, four)
However, when range()
is used in code that is part of a larger awarding, it is typically considered poor practice to utilize listing()
or tuple()
in this mode. Similar iterators, range
objects are lazy—the values in the specified range are non generated until they are requested. Using list()
or tuple()
on a range
object forces all the values to be returned at once. This is rarely necessary, and if the list is long, it tin can waste time and memory.
range(<begin>, <end>, <stride>)
returns an iterable that yields integers starting with <begin>
, upward to just non including <end>
. If specified, <stride>
indicates an amount to skip betwixt values (analogous to the stride value used for cord and list slicing):
>>>
>>> list ( range ( v , 20 , 3 )) [5, 8, xi, 14, 17]
If <stride>
is omitted, information technology defaults to 1
:
>>>
>>> list ( range ( five , ten , 1 )) [five, 6, 7, 8, 9] >>> list ( range ( 5 , 10 )) [5, 6, 7, 8, 9]
All the parameters specified to range()
must be integers, just any of them can exist negative. Naturally, if <begin>
is greater than <cease>
, <stride>
must exist negative (if you want whatever results):
>>>
>>> listing ( range ( - v , v )) [-5, -four, -3, -2, -one, 0, 1, two, three, iv] >>> list ( range ( 5 , - v )) [] >>> list ( range ( 5 , - 5 , - 1 )) [5, 4, iii, two, one, 0, -1, -ii, -three, -four]
Altering for
Loop Behavior
You saw in the previous tutorial in this introductory series how execution of a while
loop can be interrupted with suspension
and keep
statements and modified with an else
clause. These capabilities are available with the for
loop every bit well.
The break
and continue
Statements
suspension
and continue
work the aforementioned way with for
loops as with while
loops. break
terminates the loop completely and proceeds to the first statement following the loop:
>>>
>>> for i in [ 'foo' , 'bar' , 'baz' , 'qux' ]: ... if 'b' in i : ... break ... print ( i ) ... foo
proceed
terminates the current iteration and proceeds to the next iteration:
>>>
>>> for i in [ 'foo' , 'bar' , 'baz' , 'qux' ]: ... if 'b' in i : ... keep ... impress ( i ) ... foo qux
The else
Clause
A for
loop can have an else
clause too. The interpretation is coordinating to that of a while
loop. The else
clause will be executed if the loop terminates through exhaustion of the iterable:
>>>
>>> for i in [ 'foo' , 'bar' , 'baz' , 'qux' ]: ... print ( i ) ... else : ... impress ( 'Done.' ) # Will execute ... foo bar baz qux Done.
The else
clause won't be executed if the list is broken out of with a break
statement:
>>>
>>> for i in [ 'foo' , 'bar' , 'baz' , 'qux' ]: ... if i == 'bar' : ... suspension ... print ( i ) ... else : ... print ( 'Done.' ) # Will not execute ... foo
Decision
This tutorial presented the for
loop, the workhorse of definite iteration in Python.
You also learned almost the inner workings of iterables and iterators, two important object types that underlie definite iteration, simply also figure prominently in a wide variety of other Python code.
In the side by side two tutorials in this introductory series, you lot volition shift gears a little and explore how Python programs can interact with the user via input from the keyboard and output to the console.
Picket Now This tutorial has a related video course created by the Real Python team. Picket it together with the written tutorial to deepen your understanding: For Loops in Python (Definite Iteration)
How To Loop Through A Set In Python,
Source: https://realpython.com/python-for-loop/
Posted by: olivermandiess.blogspot.com
0 Response to "How To Loop Through A Set In Python"
Post a Comment